XML is a cross-platform, hardware and software independent,
text based markup language, which enables you to store data in a structured
format by using meaningful tags. XML stores structured data in XML documents
that are similar to databases. Notice that unlike Databases, XML
documents store data in the form of plain text, which can be used across
platforms.
<Books>
<Book bid="B001">
<Title> Understanding XML </Title>
<Price> $30 </Price>
<author>
<FirstName> Lily </FirstName>
<LastName>
Hicks <LastName>
</author>
</Book>
<Book bid="B002">
<Title> .NET Framework </Title>
<Price> $45 </Price>
<author>
<FirstName> Jasmine </FirstName>
<LastName>
Williams <LastName>
</author>
</Book>
</Books>
XmlDataDocument doc=new XmlDocument(ds);
XmlNodeReader reader=new XmlNodeReader(doc);
An XML document consists of elements and attributes. For this reason, you can access XML data programmatically. Note that XML DOM allows you to access and manipulate the elements and attributes present in an XML document programmatically.
To implement XML DOM, the .NET framework provides a set of additional classes included in the System.Xml namespace. These classes enable you to access the XML data programmatically. Some of the classes in the System.Xml namespace are as follows:
The Dataset object has a ReadXml method, which is used to read data into the DataSet from an XML file. The ReadXml method can read XML documents from the Stream, File, TextReader, and XmlReader sources. The synatx for the ReadXml method is as follows:
Consider that you want to represent "books.xml",
with a DataSet. For this you can read the "books.xml" file into a DataSet and
bind the XML document control to the DataGrid control by adding the following
code to the Load
event of the page in the .aspx
page.
private void Page_Load(object sender, System.EventArgs e)
{
DataSet ds = new DataSet();
ds.ReadXml (MapPath("books.xml"));
DataGrid1.DataSource = ds;
DataGrid1.DataBind();
}
Solution: It is pertinent to note that XSLT is the W3C specification for formatting XML documents and displaying the contents in the required format. You need to create an .xsl file by using Visual Studio .NET IDE to display the data stored in the XML document in a tabular format. An XML Web server control is used to display the contents of an XML document in Web application. You need to add an XML Web Server control from the toolbox to the Web form to display the relevant XML data in the Web application.
1. Open your Web application, and add an item XML file, in the same manner as shown before. So, now considering that you have added "books.xml" using the XML designer to your web application, let us move to next step.
2. Select Add New Item option from the project menu to open the Add New Item dialog box.
3. Select XSLT File as the template form the right pane.
4. Add a few lines of code after the second line (since two lines are automatically generated). To see this code you can download the project through the link at the end of this section.
Now that you have created the XML file with the relevant data, and also specified the style sheet for the XML file.
5. Next, you need to apply the style sheet to the XML data by adding an XML server control to the WebForm1.aspx and setting the DocumentSource and TransformSource properties to .xml file and .xsl file respectively.
Build your application and run it. The output should be as follows:
You can download this application through this link.
Solution: For this we'll add an XML Web server control and a label to display ant error message. The code for the same is written below:
using System.Data.SqlClient;
using System.Xml;
Dataset ds = new Dataset();
//Create a connection string.
String sqlconnect = "Persist Security Info=False;User ID=sa;Initial Catalog=WebShoppe;Data Source=IRDTEST-D190;";
//Create a connection object to connect to the web shoppe database
try
{
SqlConnection nwconnect = new SqlConnection(sqlconnect);
//Create a command string to select all the customers in the customerDetails table
String scommand = "Select * form customerDetails";
//Create an adapter to load the dataset
SqlDataAdapter da = new SqlDataAdapter(scommand,nwconnect);
//Fill the dataset
da.Fill(ds,"customerDetails");
}
catch
{
Label1.Text = "Error while connecting to database";
}
XmlDataDocument doc = new XmlDataDocument(ds);
Xml1.Document = doc;
doc.Save(MapPath("Customers.xml"));//This is where we are saving the data in an XML file Customers.xml
The above code will display the contents not in a tabular format, since no style sheets are attached. If you want the data to be displayed in some particular format, make your XSL file as myfile.xsl and add the following three lines of code to the above written code:
XslTransform t = new XslTransform();
t.Load(MapPath(("myfile.xsl"));
Xml1.Transform = t;
In an XML document, you specify the structure of the data by creating a DTD
or an XML schema. When you include a DTD in an XML document, the software checks
the structure of the XML document against the DTD. This process of checking the
structure of the XML document is called validating. The software
performing the task of validating is called a validating parser.
The following code defines the structure of an XML document that will store
data related to books:
<?xml
version="1.0"?><Books>
<Book bid="B001">
<Title> Understanding XML </Title>
<Price> $30 </Price>
<author>
<FirstName> Lily </FirstName>
<LastName>
Hicks <LastName>
</author>
</Book>
<Book bid="B002">
<Title> .NET Framework </Title>
<Price> $45 </Price>
<author>
<FirstName> Jasmine </FirstName>
<LastName>
Williams <LastName>
</author>
</Book>
</Books>
.NET Support for XML
The .NET Framework has extensive support for working with XML documents. IN
the .NET framework, the support for XML documents includes:
- XML namespace
- XML designer
- XML Web Server control
- XML DOM support
XML Namespace
The System.Xml namespace provides a rich set of classes for processing XML
data. The commonly used classes for working with XML data are:
- XmlTextReader: Provides forward only access to a stream of XML data and checks whether or not an XML document is well formed. This class neither creates as in-memory structure nor validates the XML document against the DTD. You can declare an object of the XmlTextReader class by including the System.Xml namespace in the application. The syntax to declare an object of this class is as follows:
It is important to note that the .xml file you pass as an argument to the
constructor of the XmlTextReader class exists in the
\WINNT\System32 folder.
- XmlTextWriter: Provides forward only way of generating streams or files containing XML data that conforms to W3C XML 1.0. If you want to declare an object of the XmlTextWriter class, you must include the System.Xml. The syntax to decare an object of this class is as follows:
Here
Response.Output represents an outgoing HTTP
response stream to which you want to send the XML data.
- XmlDocument: Provides navigating and edinting features of the nodes in an XML document tree. XmlDocument is the most frequently used class in ASP.NET applications that use XML documents. It also supports W3C XML DOM. XML DOM is an in-memory representation of an XML document. It represents data in the form of hierarchically organized object nodes and allows you to programmatically access and manipulate the elements and attributes present in an XML document.
- XmlDataDocument: Provides support for XML and relational data in W3C XML DOM. You can use this class with a dataset to provide relational and non-relational views of the same set of data. This class is primarily used when you want to access the functions of ADO.NET. The syntax to declare an object of this class is as follows:
XmlDataDocument doc=new XmlDocument(ds);
There are a number of reasons to use XmlDataDocument:
- It gives you the freedom to work with any data by using the DOM.
- There is synchronization between an XmlDatadocument and a DataSet, and any changes in one will be reflected in the other.
- When an XML document is loaded into an XmlDatadocument, the schema is preserved.
You need to
include System.Xml namespace.
- XmlPathDocument: Provides a read-only cache for XML document processing by using XSLT. This class is optimizied for XSLT processing and does not check for the conformity of W3C DOM. For this reason, you can create an instance of this class to process an XML document faster. To create an instance of the XPathDocument class, you need to include the System.Xml.XPath namespace in the application. The Syntax to declare an object of this class is as follows:
- XmlNodeReader: Provides forward-only access to the data represented by the XmlDocument or XmlDataDocument class. If you want to create an instance of the XmlNodeReader class, you need to include the System.Xml namespace. The syntax to declare an object of this class is as follows:
XmlNodeReader reader=new XmlNodeReader(doc);
- XslTransform: Provides support for a XSLT 1.0 style sheet syntax that enables you to transform an XML document by using XSL style sheets. If you want to create an instance of the XslTransform class, you need to include the System.Xml.Xsl namespace in the application. The syntax to declare an object of this class is as follows:
XML Designer
Visual Studio .NET provides the XML designer that you can use to create and edit XML documents. For example, if you need to create an XML document that contains the details of books available in an online bookstore, you need to perform the following steps by using the XML designer of Visual Studio .NET:
- Create a new ASP.NET Web application.
- Select the Add New Item option
- Select XML File as the template from the right pane. Specify the name as "books.xml" and click open.
- The XML designer is displayed. The XML designer has automatically generated a line that notifies the browser that the document being processed is an XML document, as displayed in the figure:
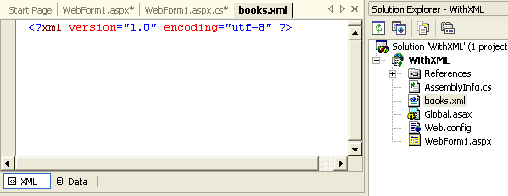
5. At the bottom of the
designer window, there are two tabs, XML and Data.
In the XML tab enter the following lines of code after the first line in
the XML designer:
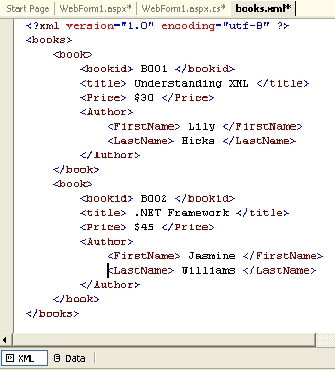
The Data view displays the XML data represented by the XML
document. When you switch to the Data view, the data appears, as displayed in
the following figure:
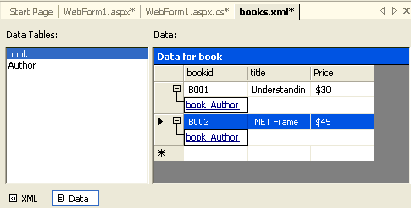
In addition to just viewing data in the Data view, you can also add data
directly to an existing XML document. For this, just click on the new row below
the existing data and enter your values, and shown in the figure:
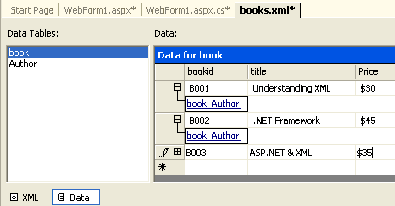
XML Web Server Control
An XML Web Server control is used to display the contents of an XML document
without formatting or using XSL Transformations. You can optionally specify a
XSLT style sheet that formats the XML document before it is displayed in an XML
server control. The XML Web Server control belongs to the
System.Web.UI.WebControls namespace. You can add an XML Web Server
control to a Web form by dragging the control from the Web forms tab of the
toolbox.
The XML Web Server control has the following properties:
- DocumentSource: Allows you to specify the URL or the path of the XML document to be displayed in the Web form.
- TransformSource: Allows you to specify the URL of the XSLT file, which transforms the XML document into the required format before it is displayed in the Web form.
- Document: Allows you to specify a reference to an object of the XMLDocument class. This property is available only at runtime.
- Transform: Allows you to specify a reference to an object of the XMLTransform class. This property is available only at runtime.
A practical example for the same is shown in the last section of this
tutorial.
XML Document Object Model Support
When you want to access and display XML data in Web Applications, you use the XML Web server control and set its properties at design time. In certain situation, you may need to display the XML data based on specific conditions. In such cases, you will have to access the XML data programmatically.An XML document consists of elements and attributes. For this reason, you can access XML data programmatically. Note that XML DOM allows you to access and manipulate the elements and attributes present in an XML document programmatically.
To implement XML DOM, the .NET framework provides a set of additional classes included in the System.Xml namespace. These classes enable you to access the XML data programmatically. Some of the classes in the System.Xml namespace are as follows:
- XmlDocumentType: Represents the DTD used by an XML document.
- XmlElement: Represents one element from an XML document.
- XmlAttribute: Represents one attribute of an element.
Bind XML Data to Web Form Controls
An XML document cannot be directly bound to server controls. To implement data binding, you first need to load the XML document into a DataSet. Then, you can bind server control with this DataSet.The Dataset object has a ReadXml method, which is used to read data into the DataSet from an XML file. The ReadXml method can read XML documents from the Stream, File, TextReader, and XmlReader sources. The synatx for the ReadXml method is as follows:
ReadXml (Stream | FileName | textReader | XmlReader ,
XmlReadMode )
The XmlReadMode
parameter can take any of the values listed
in the following table:
VALUES
|
DESCIPTION
|
Auto |
Checks the XML
document and selects the action accordingly from the following choices: 1. If the DataSet already has schema or the document contains an inline schema, it sets XmlReadMode to ReadSchema. 2. If the DataSet does not already have a schema and the document does not contain an inline schema, it sets XmlReadMode to InferSchema. |
DiffGram | Reads a Diffgram, which is a format that contains both the original and the current values of the data, and applies changes from the DiffGram to the DataSet. |
Fragment | The default namespace is read as the inline schema. |
IgnoreSchema | Ignores any inline schema and reads data into the existing DataSet schema. |
InferSchema | Ignores any inline schema and instead infers schema from the data and loads the data. |
ReadSchema | Reads any inline schema and loads the data. If the DataSet already has a schema, new tables may be added to the schema but an exception is thrown if any tables in the inline schema already exist in the DataSet. |
private void Page_Load(object sender, System.EventArgs e)
{
DataSet ds = new DataSet();
ds.ReadXml (MapPath("books.xml"));
DataGrid1.DataSource = ds;
DataGrid1.DataBind();
}
Working With XML Server Controls - Practical Example
The WebShoppe Web Site needs to create a Web application to store the details of the books available for sale in the XML format. The details of the books should be displayed in a tabular format in a browser. The details include Book Id, Title, price, First Name and Last Name of the author.Solution: It is pertinent to note that XSLT is the W3C specification for formatting XML documents and displaying the contents in the required format. You need to create an .xsl file by using Visual Studio .NET IDE to display the data stored in the XML document in a tabular format. An XML Web server control is used to display the contents of an XML document in Web application. You need to add an XML Web Server control from the toolbox to the Web form to display the relevant XML data in the Web application.
1. Open your Web application, and add an item XML file, in the same manner as shown before. So, now considering that you have added "books.xml" using the XML designer to your web application, let us move to next step.
2. Select Add New Item option from the project menu to open the Add New Item dialog box.
3. Select XSLT File as the template form the right pane.
4. Add a few lines of code after the second line (since two lines are automatically generated). To see this code you can download the project through the link at the end of this section.
Now that you have created the XML file with the relevant data, and also specified the style sheet for the XML file.
5. Next, you need to apply the style sheet to the XML data by adding an XML server control to the WebForm1.aspx and setting the DocumentSource and TransformSource properties to .xml file and .xsl file respectively.
Build your application and run it. The output should be as follows:
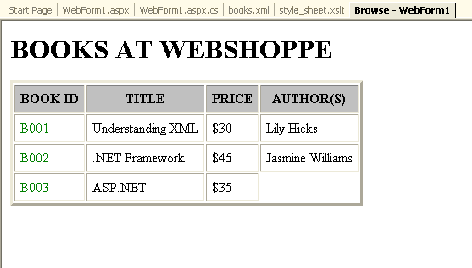
Converting Relational Data into an XML document
ASP.NET allows you to easily convert the data form a database into an Xml
document. It provides the XMLDataDocument class, which allows you to load
relational data and the data from an XML document into a DataSet. The data
loaded in XMLDataDocument can be manipulated using the W3C DOM.
Let us consider a problem: you need to extract data from a SQL Server and
store it as an XML file.Solution: For this we'll add an XML Web server control and a label to display ant error message. The code for the same is written below:
using System.Data.SqlClient;
using System.Xml;
Now add this code:
//Create
a datasetDataset ds = new Dataset();
//Create a connection string.
String sqlconnect = "Persist Security Info=False;User ID=sa;Initial Catalog=WebShoppe;Data Source=IRDTEST-D190;";
//Create a connection object to connect to the web shoppe database
try
{
SqlConnection nwconnect = new SqlConnection(sqlconnect);
//Create a command string to select all the customers in the customerDetails table
String scommand = "Select * form customerDetails";
//Create an adapter to load the dataset
SqlDataAdapter da = new SqlDataAdapter(scommand,nwconnect);
//Fill the dataset
da.Fill(ds,"customerDetails");
}
catch
{
Label1.Text = "Error while connecting to database";
}
XmlDataDocument doc = new XmlDataDocument(ds);
Xml1.Document = doc;
doc.Save(MapPath("Customers.xml"));//This is where we are saving the data in an XML file Customers.xml
The above code will display the contents not in a tabular format, since no style sheets are attached. If you want the data to be displayed in some particular format, make your XSL file as myfile.xsl and add the following three lines of code to the above written code:
XslTransform t = new XslTransform();
t.Load(MapPath(("myfile.xsl"));
Xml1.Transform = t;
06:48
|
Category:
|
0
comments